LC Hard Problem: Follow the Hints - Part II
From the get-go one can see that this problem is either a DP or BFS, likely the latter rather than the former, as confirmed by the hints. At the end it is a straightforward BFS with the only caveat of allowing for a cell that contains an obstacle IIF we still have enough "k"s to remove it. Keep an eye on the key to mark the cell as visited, noticed the formula to guarantee an unique number off of the 3-tuple {row, col, k). Code is down below, cheers, ACC.
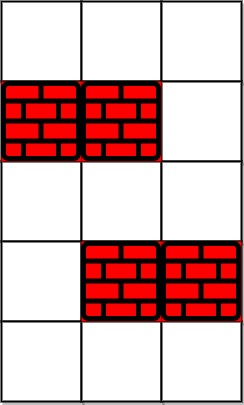
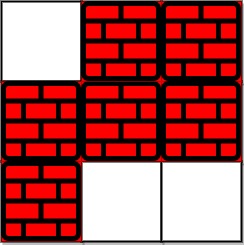
1293. Shortest Path in a Grid with Obstacles Elimination
Hard
You are given an m x n
integer matrix grid
where each cell is either 0
(empty) or 1
(obstacle). You can move up, down, left, or right from and to an empty cell in one step.
Return the minimum number of steps to walk from the upper left corner (0, 0)
to the lower right corner (m - 1, n - 1)
given that you can eliminate at most k
obstacles. If it is not possible to find such walk return -1
.
Example 1:
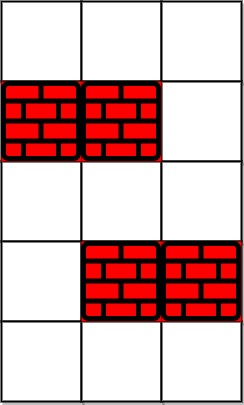
Input: grid = [[0,0,0],[1,1,0],[0,0,0],[0,1,1],[0,0,0]], k = 1 Output: 6 Explanation: The shortest path without eliminating any obstacle is 10. The shortest path with one obstacle elimination at position (3,2) is 6. Such path is (0,0) -> (0,1) -> (0,2) -> (1,2) -> (2,2) -> (3,2) -> (4,2).
Example 2:
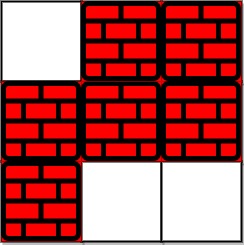
Input: grid = [[0,1,1],[1,1,1],[1,0,0]], k = 1 Output: -1 Explanation: We need to eliminate at least two obstacles to find such a walk.
Constraints:
m == grid.length
n == grid[i].length
1 <= m, n <= 40
1 <= k <= m * n
grid[i][j]
is either0
or1
.grid[0][0] == grid[m - 1][n - 1] == 0
Accepted
124,222
Submissions
284,936
public class GridObstacle { public int row; public int col; public int k; public int steps; public long key; public GridObstacle(int row, int col, int k, int steps) { this.row = row; this.col = col; this.k = k; this.steps = steps; key = (long)k * 2000 + row * 42 + col; } } public int ShortestPath(int[][] grid, int k) { Queuequeue = new Queue (); Hashtable visited = new Hashtable(); GridObstacle go = new GridObstacle(0, 0, grid[0][0], 0); visited.Add(go.key, true); queue.Enqueue(go); while (queue.Count > 0) { GridObstacle currentGo = queue.Dequeue(); if (currentGo.row == grid.Length - 1 && currentGo.col == grid[0].Length - 1) return currentGo.steps; int[] rowDelta = { 1, -1, 0, 0 }; int[] colDelta = { 0, 0, 1, -1 }; for (int i = 0; i < rowDelta.Length; i++) { int row = currentGo.row + rowDelta[i]; int col = currentGo.col + colDelta[i]; if (row >= 0 && row < grid.Length && col >= 0 && col < grid[row].Length) { GridObstacle newGo = null; if (grid[row][col] == 0) { newGo = new GridObstacle(row, col, currentGo.k, currentGo.steps + 1); if (!visited.ContainsKey(newGo.key)) { visited.Add(newGo.key, true); queue.Enqueue(newGo); } } else { if (currentGo.k < k) { newGo = new GridObstacle(row, col, currentGo.k + 1, currentGo.steps + 1); if (!visited.ContainsKey(newGo.key)) { visited.Add(newGo.key, true); queue.Enqueue(newGo); } } } } } } return -1; }
Comments
Post a Comment